Understanding Variable Types in NinjaTrader 8 Trading Strategies [Lesson 2]
In this post, we will dive deeper into the topic of variable types, which are essential building blocks for developing robust and dynamic trading strategies and indicators in NinjaTrader 8.
![Understanding Variable Types in NinjaTrader 8 Trading Strategies [Lesson 2]](/content/images/size/w2000/2023/06/Understanding-Variable-Types.jpg)
Introduction
Welcome back to our blog series on C# programming for NinjaTrader 8 trading strategies and indicators! In the previous post, we introduced the advantages of learning C# programming for traders and explored how coding skills empower traders to test their ideas and leverage complex indicators. In this post, we will dive deeper into the topic of variable types, which are essential building blocks for developing robust and dynamic trading strategies and indicators in NinjaTrader 8.
What is a Variable?
Before we dive into variable types, let's understand what a variable is. In programming, a variable is a named container that holds a value. Think of it as a labelled box where you can store and retrieve data. Variables are used to store different types of information, such as numbers, text, or logical values, and they allow us to manipulate and work with data in our programs.
Variables serve several important purposes in trading strategies. They enable us to store and update critical information like prices, indicators values, or trading signals values. By using variables, we can perform calculations, make decisions based on specific conditions, and control the behaviour of our trading strategies dynamically.
Now that we have a clear understanding of what variables are, let's explore the fundamental variable types used in NinjaTrader 8 trading strategies.
Bool Variables:
- Bool variables are used to represent true or false values.
- In trading strategies, bool variables are often used to control the execution of specific conditions or actions.
- For example, a bool variable can be used to determine whether a trade should be placed based on a certain trading signal.
Double Variables:
- Double variables are used to store decimal numbers with a higher degree of precision.
- In trading strategies, double variables are commonly used to represent price values, indicators values, or calculated metrics.
- For example, a double variable can store the value of a moving average or the profit target for a trade.
Int Variables:
- Int variables are used to store whole numbers without decimal places.
- In trading strategies, int variables are often used to represent quantities, such as the number of contracts or shares to trade.
- Additionally, int variables can be used for counting occurrences or iterations in loops.
Other Types of Variables
In addition to the bool, double, and int variable types we discussed earlier, there are other types of variables that you may encounter when working with NinjaTrader 8 or C# programming in general. Some of these include:
- String: Used to store text or characters. Strings are commonly used for displaying information, labelling variables, or working with textual data.
- DateTime: Used to represent date and time values. DateTime variables are useful when dealing with time-based calculations or when recording timestamps for trading events.
- Enum: Short for "enumeration," an enum is a custom variable type that represents a set of named values. Enums provide a convenient way to define a limited set of options or choices.
Practical Examples
Now, we will delve into the practical aspect of variable types in NinjaTrader 8 strategies and indicators. Understanding how to utilize bool, double, and int variables is essential for developing effective trading tools. Let's explore some practical examples to solidify our understanding.
First, let's create a new simple indicator with no input parameters and with one plot, just to make something practical. If you don't know how to create an indicator, you can follow our tutorial Getting Started with NinjaScript Editor: Creating Your First Basic Indicator, and once you've learned how to create a basic indicator, you can come back here.
When you are done, it should look similar to this.
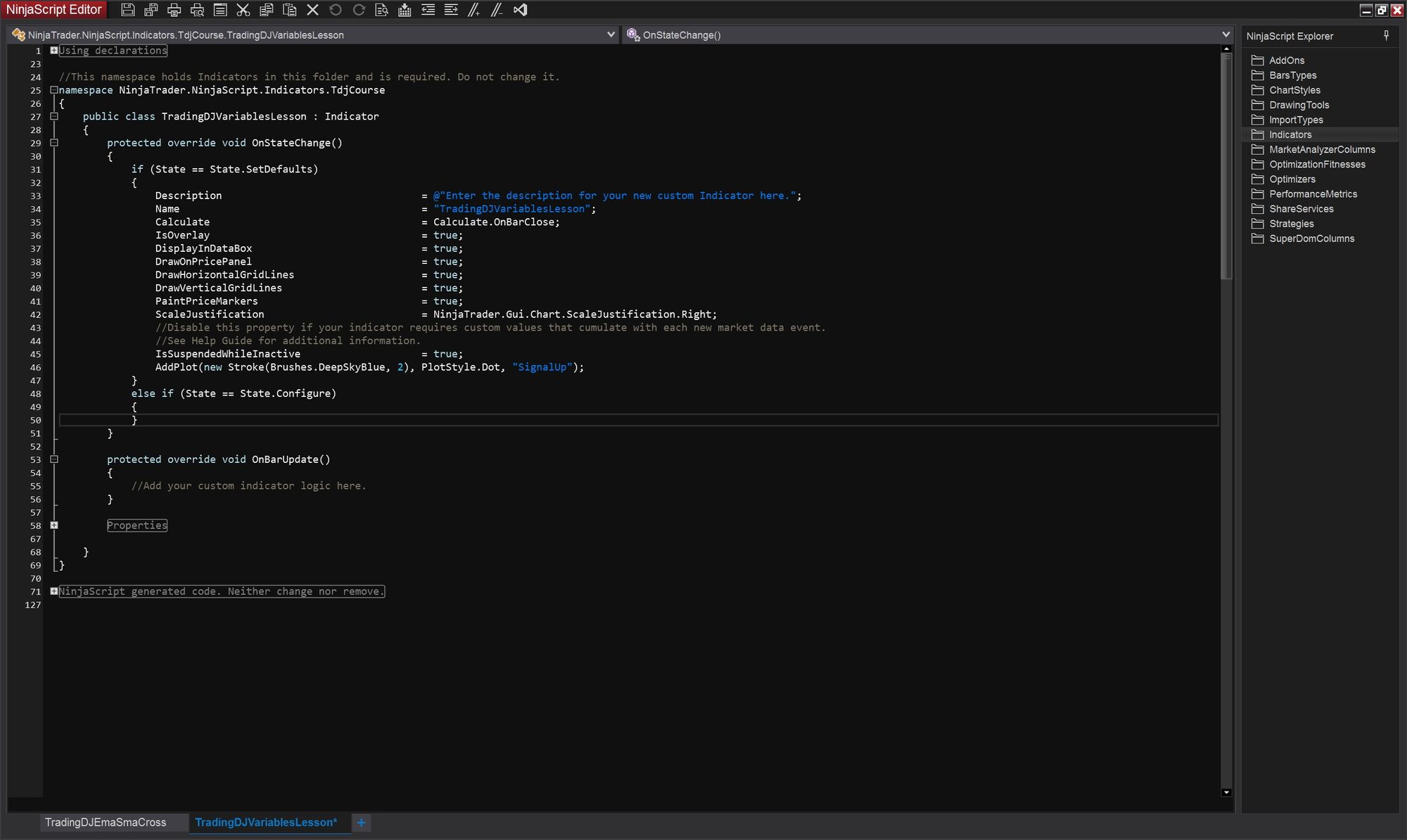
Example 1: Using bool Variables
Suppose we want to create a trading tool that performs a specific action when a condition is met. We can declare a bool variable called "isConditionMet" and set it to true when the condition is satisfied. We will add this logic in the OnStateChange Method.
In C#, a method begins and ends with a pair of curly braces. You can refer to this as the opening and closing curly braces of the method.For example:
public void MyMethod()
{
// Method implementation goes here
// ...
}
In this case, the opening curly brace ({) signifies the start of the method, and the closing curly brace (}) marks the end of the method. The code within the curly braces defines the scope and contents of the method.
We are going to add this code snippet in the OnStateChange method:
else if (State == State.DataLoaded)
{
bool isConditionMet = false;
// Perform your calculations and determine the condition
// isConditionMet = true;
// Just a side note, when you use two forward slashed "//" in C#, anything
// after the slashes will be considered a comment
if (isConditionMet)
{
Print("Condition is met! Custom message.");
}
}
We declared a variable called isConditionMet
and assigned a false
value to it. Then we added an if statement (we will learn more about it in our future lessons) and if this variable is true, the indicator will print the message "Condition is met! Custom message."
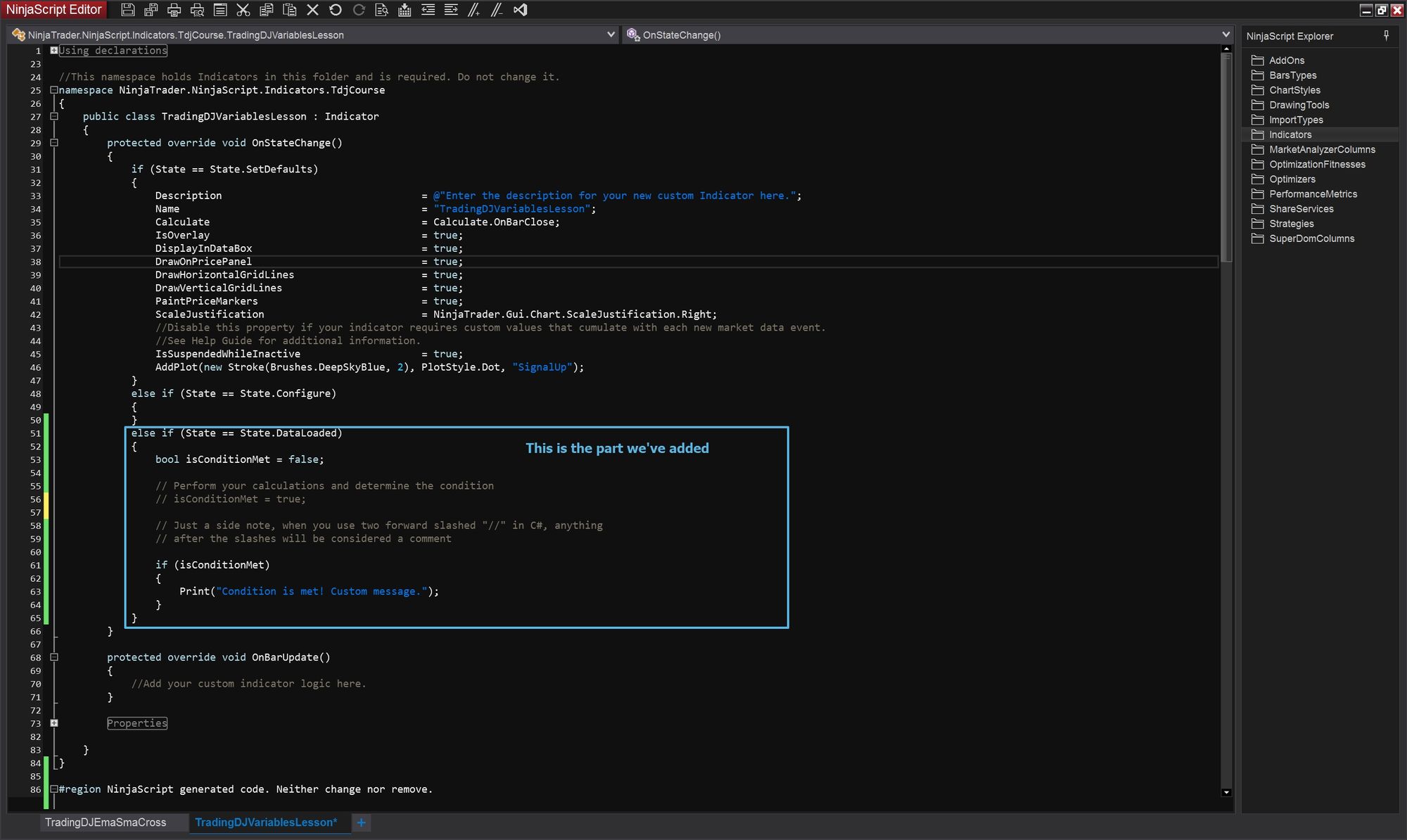
Now you can compile the indicator, add it to a chart and check the "NinjaScript Output".
To open the NinjaScript Output window, you can go to the "New" menu in the Control Center and select "NinjaScript Output."
To assign true
to the isConditionMet
variable, simply uncomment the line, compile again and refresh the chart.
// isConditionMet = true;
To uncomment it, you would remove the "//" at the beginning:
isConditionMet = true;
Here is what you should see.
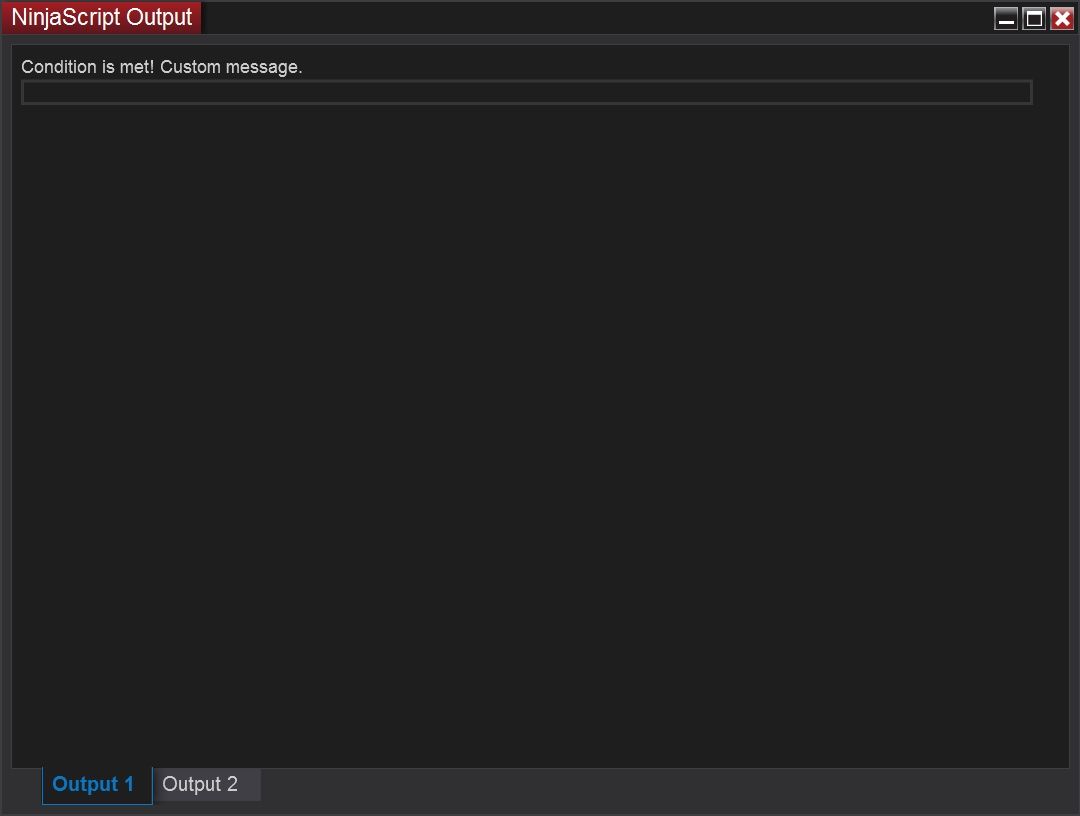
Example 2: Working with double and int Variables
Let's consider a scenario where we want to calculate the average price of an instrument over a specific period. We can use double and int variables to track the total price and the number of data points considered. Here's an example implementation within the OnStateChange method (we left the bool part):
else if (State == State.DataLoaded)
{
bool isConditionMet = false;
// Perform your calculations and determine the condition
isConditionMet = true;
// Just a side note, when you use two forward slashed "//" in C#, anything
// after the slashes will be considered a comment
if (isConditionMet)
{
Print("Condition is met! Custom message.");
}
//Here we start working with int and double type
Print("Working with int and double");
double totalPrice = 0.0;
int dataPoints = 0;
// Iterate through the data series and accumulate the prices
// ...
//first iteration
totalPrice = totalPrice + 10;
dataPoints = dataPoints + 1;
//second iteration
totalPrice = totalPrice + 20;
dataPoints = dataPoints + 1;
//third iteration
totalPrice = totalPrice + 15;
dataPoints = dataPoints + 1;
double averagePrice = totalPrice / dataPoints;
Print("Average Price: " + averagePrice);
}
Remember, these are simplified examples to illustrate the use of bool, double, and int variables in NinjaTrader indicators. You can adapt and expand upon these concepts based on your specific trading strategies and requirements.
For a visual guide and step-by-step instructions, refer to the screenshots provided below:
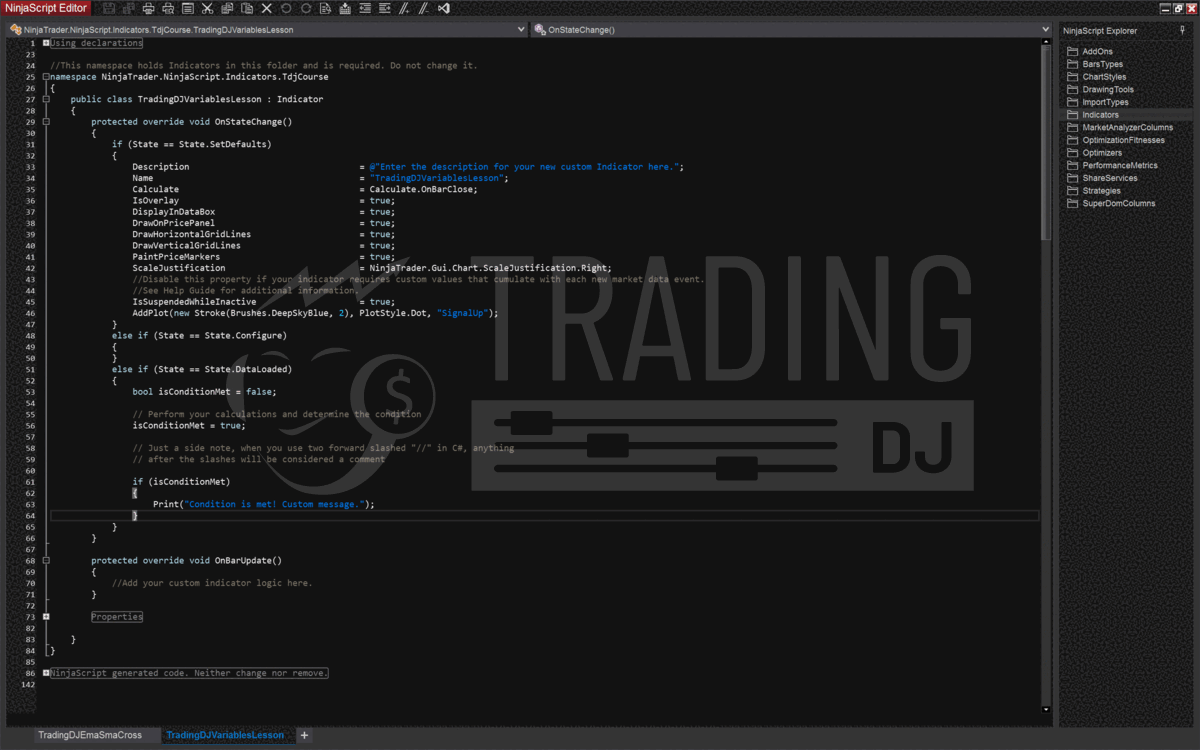
Updating the OnBarUpdate Method
To demonstrate the practical application of variable types, let's enhance our indicator logic by updating the OnBarUpdate method. By adding additional conditions and logic, we can create a more robust and customized trading tool. Below is an example of the updated method:
protected override void OnBarUpdate()
{
// Ensure we have enough bars loaded
if (CurrentBar < 2)
return;
// Determine the prior high
double priorHigh = Math.Max(High[1], High[2]);
// Check the first condition
bool firstConditionMet = Close[0] > priorHigh;
// Check the second condition
bool secondConditionMet = Low[0] > EMA(10)[0];
// Combine the conditions and take action
if (firstConditionMet && secondConditionMet)
{
SignalUp[0] = Low[0] - 5 * TickSize;
}
// Add any necessary additional logic or conditions here
// Add more code to further customize your indicator's behavior
// End of OnBarUpdate method
}
In the updated OnBarUpdate method, we introduced two additional conditions, firstConditionMet
and secondConditionMet
. The priorHigh
variable is calculated as the highest high of the last two bars using the Math.Max
function. If both conditions are met, we take action by assigning a value to the SignalUp
series.
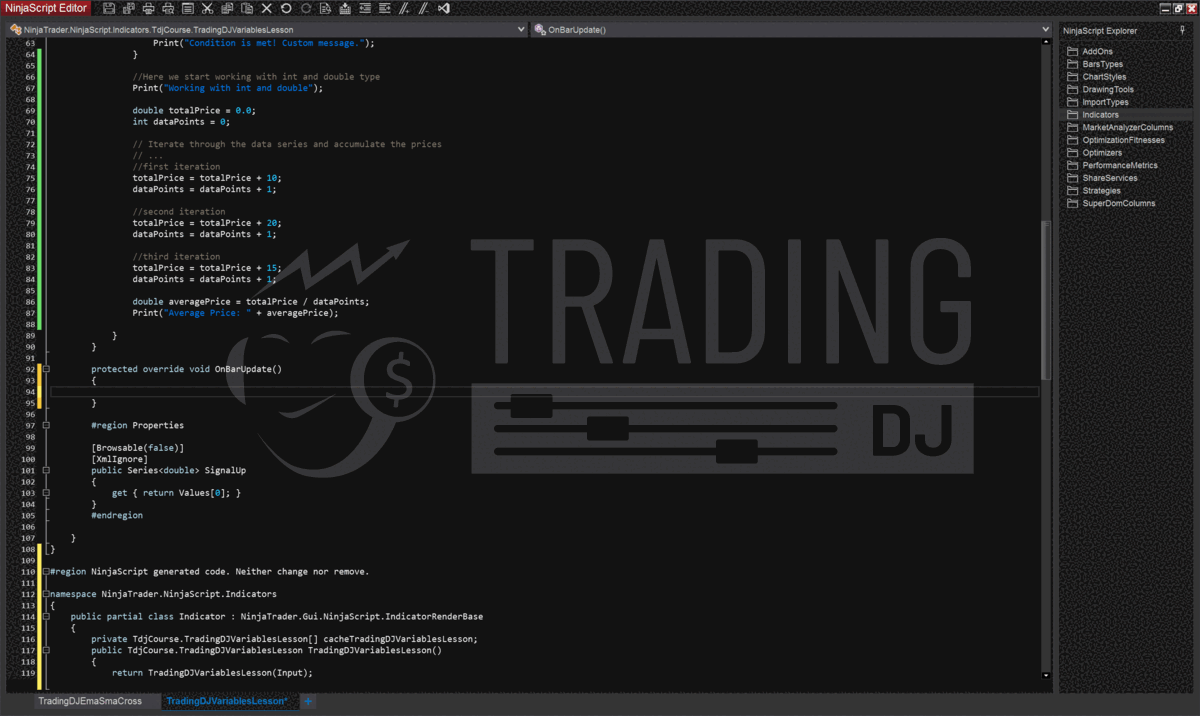
Feel free to experiment and add more conditions or customize the logic to match your trading strategy. Comments within the code help explain the purpose and functionality of each section, making it easier to understand and modify the code as needed.
Custom Variables
Apart from the built-in variable types, C# allows you to create your own custom variables using classes. Custom variables, also known as objects, are instances of user-defined classes. By defining a custom class, you can encapsulate multiple variables and functions into a single entity, representing more complex concepts or structures within your trading strategies.
Custom variables offer flexibility and allow you to model specific entities related to trading, such as financial instruments, positions, or trading rules. By defining the properties (variables) and methods (functions) within your custom class, you can create powerful and expressive representations of these entities in your code.
Download
In addition, if you want to explore the example described in this blog post hands-on, you can download the zip file named "TdjCourseVariableTypes." This zip file contains the complete code for the indicator we built during this tutorial.
To import the zip file into NinjaTrader, follow these steps:
- Open NinjaTrader and go to the "Tools" menu.
- Select "Import" from the drop-down menu.
- Choose "NinjaScript Add-On" from the options.
- Locate and select the "TdjCourseVariableTypes.zip" file you downloaded.
- Click "OK" to import the add-on.
Once imported, you will have access to the indicator code, which you can study, modify, and use as a reference for your own trading strategies. This hands-on experience will further enhance your understanding of variable types and their practical implementation in NinjaTrader 8.
Conclusion
In this blog post, we explored the fundamental variable types used in NinjaTrader 8 trading strategies, including bool, double, and int. We discussed their characteristics, how to declare and initialize variables, and provided practical examples to showcase their usage in a simple NinjaTrader indicator.
By applying these variable types in our indicator, we were able to create conditions based on price levels, moving averages, and prior highs. This allowed us to implement specific trading logic and take action when certain conditions were met.
Furthermore, we introduced the concept of custom variables, which enables you to define your own variables with custom data types using classes. This gives you the flexibility to create complex data structures and encapsulate related information within your trading strategies.
Understanding variable types and their applications, as well as the potential of custom variables, will empower you to effectively control trading logic, handle various data types, and create expressive representations of real-world entities in your trading strategies.
In the next blog post, we will delve into operators and their role in performing mathematical calculations, making logical comparisons, and manipulating variables within your trading strategies. Stay tuned for more exciting insights and practical examples!
Note: If you haven't already, make sure to check out the previous parts of our blog series, where we cover the basics of setting up NinjaTrader 8 and creating your first indicator.