Operators and Expressions in NinjaTrader 8 Trading Strategies [Lesson 3]
Operators are symbols that perform specific operations on variables and values, allowing you to manipulate and compare data within your trading strategies and indicators. Understanding operators is essential for building powerful and dynamic trading logic.
![Operators and Expressions in NinjaTrader 8 Trading Strategies [Lesson 3]](/content/images/size/w2000/2023/06/NinjaTrader-programming-course---operators.jpg)
Introduction
In the previous blog post, we explored the fundamental variable types used in NinjaTrader 8 trading strategies. Now, let's dive into the world of operators and expressions. Operators are symbols that perform specific operations on variables and values, allowing you to manipulate and compare data within your trading strategies and indicators. Understanding operators is essential for building powerful and dynamic trading logic. So, let's get started!
Arithmetic Operators
Arithmetic operators are used to perform mathematical calculations on variables and values. Here are some commonly used arithmetic operators:
- Addition (+): Adds two values together.
- Subtraction (-): Subtracts one value from another.
- Multiplication (*): Multiplies two values.
- Division (/): Divides one value by another.
- Modulus (%): Returns the remainder after division.
Let's consider an example where we calculate the average of the last three closing prices:
double average = (Close[0] + Close[1] + Close[2]) / 3;
The +
operator adds the three closing prices together, and the /
operator divides the sum by 3 to calculate the average. You can then use the average
variable in your trading logic.
Comparison Operators
Comparison operators are used to compare variables and values, returning a boolean result (true
or false
). Here are some common comparison operators:
- Equal to (==): Checks if two values are equal.
- Not equal to (!=): Checks if two values are not equal.
- Greater than (>): Checks if one value is greater than another.
- Less than (<): Checks if one value is less than another.
- Greater than or equal to (>=): Checks if one value is greater than or equal to another.
- Less than or equal to (<=): Checks if one value is less than or equal to another.
Let's look at examples where we compare the current closing price with the previous closing price:
bool isPriceIncreasing = Close[0] > Close[1];
The >
operator compares the current closing price (Close[0]
) with the previous closing price (Close[1]
). The result is stored in the isPriceIncreasing
variable, indicating whether the price is increasing (true
) or not (false
).
bool isPriceNotEqual = Close[0] != Close[1];
The !=
operator is used to check if two values are not equal. For example, you can compare the current closing price with the previous closing price to determine if they are not equal.
Logical Operators
Logical operators are used to combine and manipulate boolean values. Here are the three main logical operators:
- AND (&&): Returns true if both expressions are true.
- OR (||): Returns true if at least one expression is true.
- NOT (!): Reverses the boolean value of an expression.
Consider a scenario where you want to check if the current price is above a certain threshold and if the volume is above a specific level:
bool isPriceAboveThreshold = Close[0] > threshold;
bool isVolumeAboveLevel = Volume[0] > volumeLevel;
bool isConditionMet = isPriceAboveThreshold && isVolumeAboveLevel;
Here, we use the &&
(logical AND) operator to check if both conditions are true. The isConditionMet
variable will be true
only if both isPriceAboveThreshold
and isVolumeAboveLevel
are true
.
Conditional Operator (Ternary Operator)
The conditional operator, also known as the ternary operator, allows you to assign a value to a variable based on a condition. Let's say you want to determine the direction of a signal based on whether the current price is above a threshold:
double signal = (Close[0] > threshold) ? 1.0 : -1.0;
If the condition (Close[0] > threshold)
is true, the value 1.0
is assigned to the signal
variable. Otherwise, the value -1.0
is assigned.
OnBarUpdate Method with Examples
Here's an example OnBarUpdate
method that incorporates the operators we discussed:
protected override void OnBarUpdate()
{
// Ensure we have enough bars loaded
if (CurrentBar < 2)
return;
// Print a divider for each bar
Print("------------------------");
Print("Time: " + Time[0]);
// Arithmetic operator example
double average = (Close[0] + Close[1] + Close[2]) / 3;
Print("Average Close: " + average);
// Comparison operator example
bool isPriceIncreasing = Close[0] > Close[1];
Print("Is Price Increasing? " + isPriceIncreasing);
// Logical operator example
double threshold = average;
double volumeLevel = (Volume[0] + Volume[1] + Volume[2]) / 3;
bool isPriceAboveThreshold = Close[0] > threshold;
bool isVolumeAboveLevel = Volume[0] > volumeLevel;
bool isConditionMet = isPriceAboveThreshold && isVolumeAboveLevel;
Print("Is Condition Met? " + isConditionMet);
// Conditional operator example
double signal = (Close[0] > threshold) ? 1.0 : -1.0;
Print("Signal: " + signal);
// Example with != operator
bool isPriceNotEqual = Close[0] != Close[1];
Print("Is Price Not Equal? " + isPriceNotEqual);
}
Here is the output we see in the NinjaScript Output:
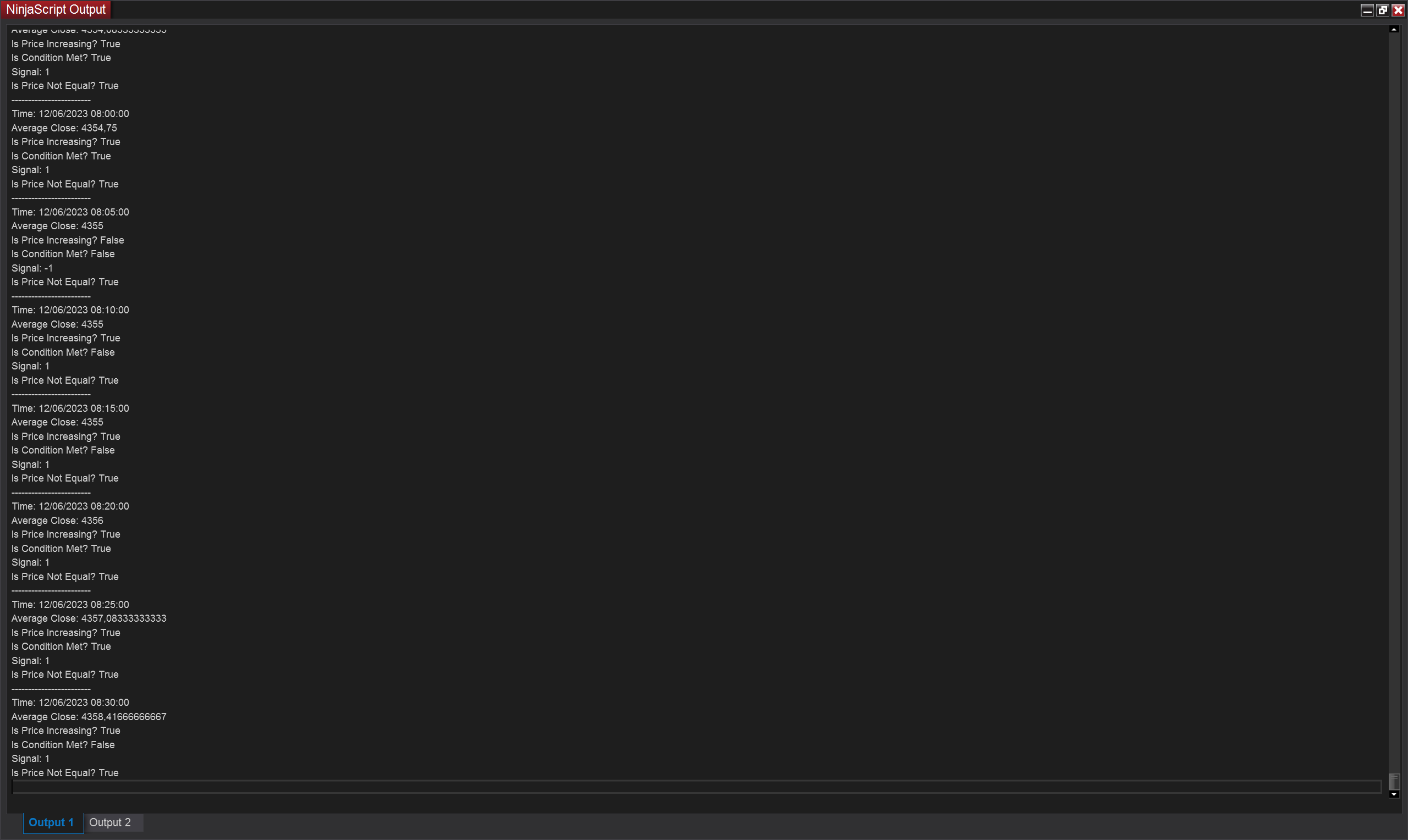
By experimenting with these examples and understanding the power of operators and expressions, you can create sophisticated trading strategies tailored to your specific needs.
Feel free to download the complete code examples discussed in this blog post from the TdjCourseOperators.zip file. Import the file in NinjaTrader using the "Tools" > "Import" > "NinjaScript Add-On" menu option.
In the next blog post, we will explore control structures, such as if-else statements and loops, to further enhance your trading strategies and indicators. Stay tuned for more insights and practical examples!