How to Make a Sound Alert in NinjaTrader 8 (Step-by-Step)
Learn how to add sound alerts in NinjaTrader 8 using PlaySound, with user-configurable options and real trading strategy examples based on TradingDJ indicators.
1) Introduction & Why It Matters
Sound alerts are a simple but powerful way to stay responsive to market conditions—especially when you're multitasking or trading multiple instruments. In NinjaTrader 8, it's easy to add basic audio notifications using PlaySound()
. But if you want full control—custom sound files, user parameters, and smarter logic—you’ll need to go a step further.
This tutorial starts with the basics and moves into a more advanced implementation you can use in your custom indicators and TradingDJ strategies.
2) Basic Alert Using PlaySound()
If you just want a quick sound when something happens, NinjaTrader provides a built-in method:
PlaySound(@"C:\mySound.wav");
This will play a sound file from a specific path. You can also use one of the default sounds that come with NinjaTrader:
PlaySound(NinjaTrader.Core.Globals.InstallDir + @"\sounds\Alert1.wav");
These sounds are located in:
C:\Program Files (x86)\NinjaTrader 8\sounds\
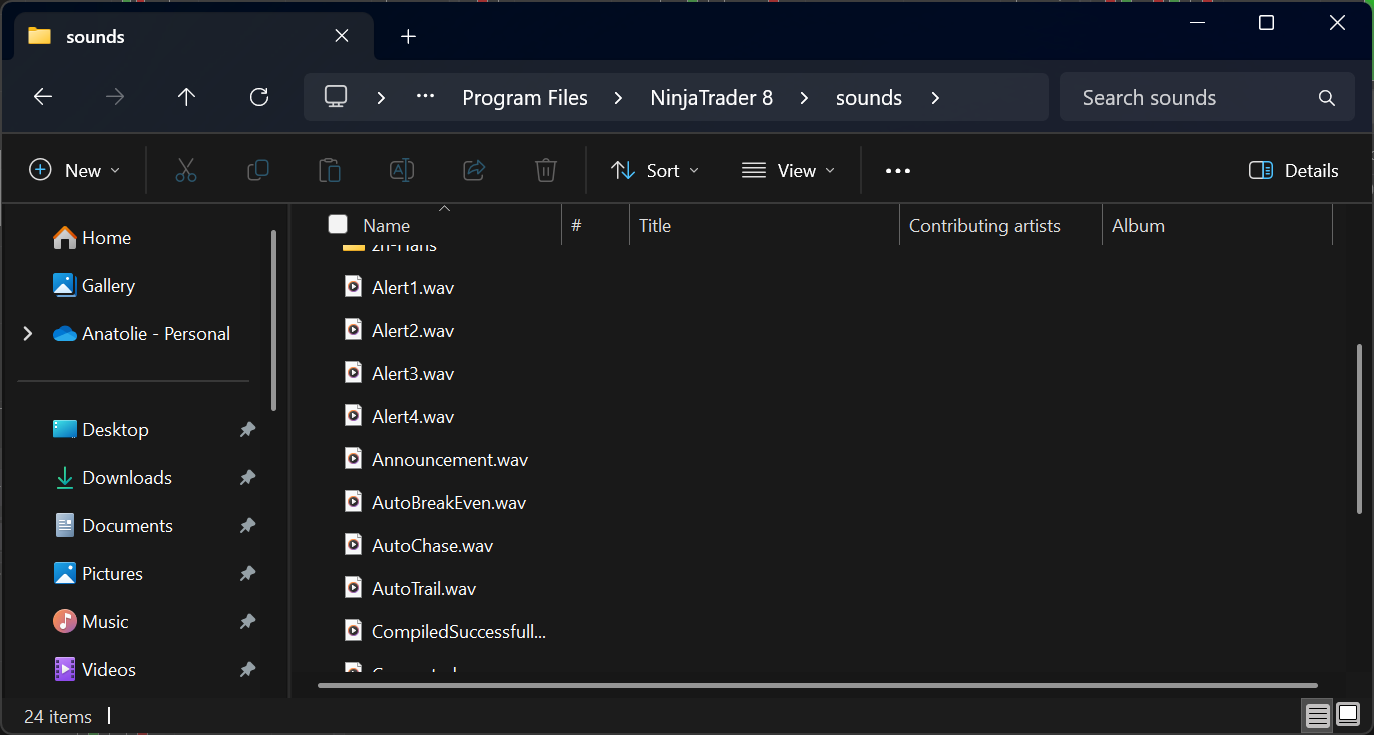
Use this inside OnBarUpdate()
or any conditional block. For example:
if (Close[0] > Open[0])
{
PlaySound(NinjaTrader.Core.Globals.InstallDir + @"\sounds\Alert1.wav");
}
Simple, clean, and works well for quick alerts.
3) Adding Parameters for Custom Sound Alerts
To give users control over when and what sound plays, you can add two public parameters to your indicator or strategy:
[NinjaScriptProperty]
[Display(Name = "Sound Alert", Order = 4, GroupName = "Parameters")]
public bool EnableSoundAlert { get; set; }
[NinjaScriptProperty]
[Display(Name = "Sound File", Order = 5, GroupName = "Parameters")]
public string SoundAlertFile { get; set; }
And set the defaults in the State.SetDefaults
phase:
if (State == State.SetDefaults)
{
EnableSoundAlert = false;
SoundAlertFile = "Alert1.wav";
}
In your logic, you'll use a private variable to store the resolved path:
private string soundFilePath;
Now initialize the path after the State.DataLoaded
stage:
else if (State == State.DataLoaded)
{
initSoundFiles();
}
Here’s the method that handles it:
private void initSoundFiles()
{
if (SoundAlertFile.Contains("/") || SoundAlertFile.Contains("\\"))
{
soundFilePath = SoundAlertFile;
}
else
{
soundFilePath = NinjaTrader.Core.Globals.InstallDir + @"\sounds\" + SoundAlertFile;
}
}
Then call your alert logic like this:
private void manageSoundAlert()
{
if ((SignalUp[0] || SignalDown[0]) && EnableSoundAlert)
{
PlaySound(soundFilePath);
}
}
This setup gives you flexibility—users can select a file in NinjaTrader’s default sounds folder or enter a full path to any .wav
file on their system.
4) Strategy Integration Example with a TradingDJ Indicator
Let’s bring everything together in a real-world TradingDJ strategy.
Suppose you're using the Alternating Candle Colors + Donchian Range Filter setup described in this article. In some cases, you might want to trigger a sound alert when a signal appears, but the range filter is not OK—for instance, to monitor potential entries that are filtered out.
Here’s how you could implement that:
Simple Version (hardcoded sound)
private void manageSoundAlert()
{
if ((alternatingBodyColor.SignalUp[0] || alternatingBodyColor.SignalDown[0])
&& !rangeFilterOk[0])
{
PlaySound(NinjaTrader.Core.Globals.InstallDir + @"\sounds\Alert1.wav");
}
}
This version uses a fixed sound file from the NinjaTrader default sounds directory.
Parameterized Version (user-configurable)
private void manageSoundAlert()
{
if ((alternatingBodyColor.SignalUp[0] || alternatingBodyColor.SignalDown[0])
&& !rangeFilterOk[0] && EnableSoundAlert)
{
PlaySound(soundFilePath);
}
}
In this version, the alert:
- Only triggers when
EnableSoundAlert
is set totrue
. - Plays a custom file defined by the user through the
SoundAlertFile
parameter.
This approach gives traders more flexibility and control, especially when monitoring different strategies across instruments or timeframes.
Download
You can download the complete example below, or see it on GitHub.
5) Wrap-Up
Sound alerts help you catch key moments without staring at charts all day. NinjaTrader makes it easy to start, and with just a few extra lines, you can offer powerful alerting features in your indicators or strategies.
Try This Now:
Add PlaySound(NinjaTrader.Core.Globals.InstallDir + @"\sounds\Alert1.wav")
to your existing logic.
Then extend it using EnableSoundAlert
and SoundAlertFile
parameters.
Finally, integrate it into your TradingDJ strategies for smarter notifications.
Let the market talk to you—literally.