Adding Parameters to your NinjaTrader 8 Indicator
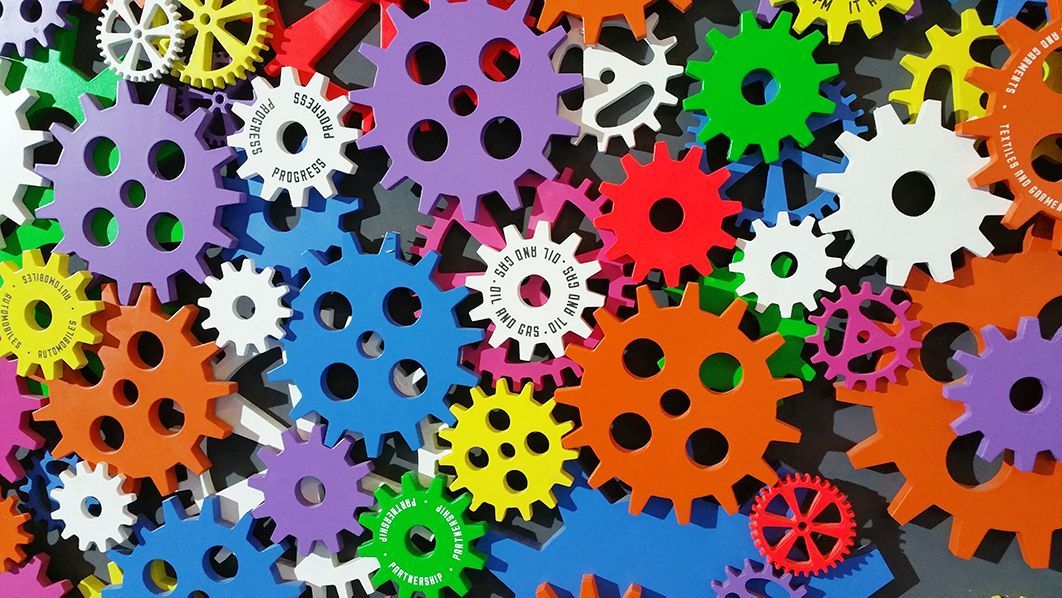
In the previous article, we created a NinjaTrader 8 indicator. The goal was to write a simple Inside Bar Indicator preceded by three bars that make consecutive lower lows and lower highs or consecutive higher lows and higher highs.
What if we decided to modify it a little bit, by adding a parameter that allows changing the number of consecutive bars preceding the Inside Bar?
Do you remember that we talked about different ways of writing an indicator or strategy?
bool isIBPrecededByFallingBars = High[0] <= High[1] && Low[0] >= Low[1]
&& High[1] < High[2] && Low[1] < Low[2]
&& High[2] < High[3] && Low[2] < Low[3]
&& High[3] < High[4] && Low[3] < Low[4];
Instead, to complete our task, we have to add a parameter to our indicator because we have already added a constant that keeps the value of the minimum consecutive rising or falling bars preceding the inside bar.
//The number of minimum consecutive rising or falling bars preceding the inside bar
private const int minimumConsecutiveBars = 3;
Nevertheless, let's start to create a new indicator from the beginning. This way, the wizard will create the parameter for ourselves.
Creating the indicator using the Wizard
First, we need to open the NinjaScript Editor (New -> NinjaScript Editor).
Then right-click the Indicators on the Indicators folder in the NinjaScript Editor and choose New Indicator.
Using the New Indicator Wizard, we will enter the values as follows:
General Page:
Name TradingDJInsideBarParameter
Description A simple Inside Bar Indicator preceded by N bars that make consecutive lower lows and lower highs or consecutive higher lows and higher highs. We will use the parameter MinimumConsecutiveBars to keep the minimum number of bars.
Default Properties Page:
Calculate: On bar close
Overlay on price: Checked
More properties: Uncheck all checkboxes
Additional Data Page:
Leave all empty
Additional Event Methods Page:
Leave all checkboxes unchecked
Input Parameters Page:
Click add to add a new parameter with the following details.
Name MinimumConsecutiveBars
Type int
Default 3
Min 0
Description The number of minimum consecutive rising or falling bars preceding the inside bar
Plots and Lines Page:
Leave empty
Once done, click Finish to see the plain Indicator tab opened in the editor.
The wizard produced the following code in our Properties region.
#region Properties
[NinjaScriptProperty]
[Range(0, int.MaxValue)]
[Display(Name="MinimumConsecutiveBars", Description="The number of minimum consecutive rising or falling bars preceding the inside bar", Order=1, GroupName="Parameters")]
public int MinimumConsecutiveBars
{ get; set; }
#endregion
Indeed we could only add it to the previous indicator instead of starting to write a new one.
Importing the logic of the indicator
Now we can get the logic of the indicator we had written in the previous post.
Let's copy the variables for keeping the number of rising and falling bars and le'ts get rid of the constant minimumConsecutiveBars, we will use the added parameter for checking the if the number or falling or rising bars respects our minimum.
#region Variables
//Will keep the number of rising bars
private int risingBarsCount;
//Will keep the number of falling bars
private int fallingBarsCount;
#endregion
Then we will have to initialize these variables in the OnStateChange method.
...
else if (State == State.Configure)
{
risingBarsCount = 0;
fallingBarsCount = 0;
}
...
Now we can copy (we will have to adjust the name of the variable minimumConsecutiveBars -> MinimumConsecutiveBars) the methods checkForInsideBar(), manageRisingBars() and manageFallingBars() and then we can call these methods inside OnBarUpdate().
protected override void OnBarUpdate()
{
// Since the calculations require that there be at least one previous bar
// we'll need to check for that and exit if this is the first bar.
if (CurrentBar < 1)
{
return;
}
checkForInsideBar();
manageRisingBars();
manageFallingBars();
}
private void checkForInsideBar()
{
if(risingBarsCount >= MinimumConsecutiveBars
&& High[0] <= High[1] && Low[0] >= Low[1])
{
//Here goes the logic for the pattern Inside Bar and minimumConsecutiveBars rising bars
//We will simply set the color of the inside bar orange
BarBrush = Brushes.Orange;
}
if(fallingBarsCount >= MinimumConsecutiveBars
&& High[0] <= High[1] && Low[0] >= Low[1])
{
//Here goes the logic for the pattern Inside Bar and minimumConsecutiveBars falling bars
//We will simply set the color of the inside bar blue
BarBrush = Brushes.Blue;
}
}
private void manageRisingBars()
{
if(High[0] > High[1] && Low[0] > Low[1])
{
risingBarsCount++;
}
else
{
risingBarsCount = 0;
}
}
private void manageFallingBars()
{
if(High[0] < High[1] && Low[0] < Low[1])
{
fallingBarsCount++;
}
else
{
fallingBarsCount=0;
}
}
Once compiled, we can add the new indicator to the chart, to see if it is working correctly. We can change the number consecutive number needed for the inside bar by changing the parameter we added.
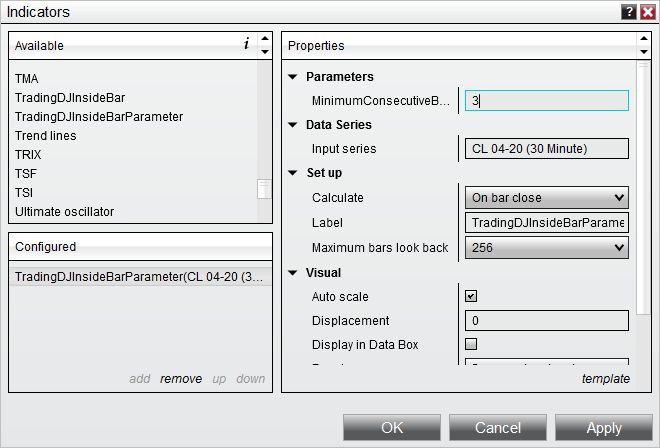
By setting MinimumConsecutiveBars to 0, the indicator will color every inside bar on the chart. You can play with this parameter to see what you get.
Here are two examples, setting MinimumConsecutiveBars to 4 and then setting it to 1.
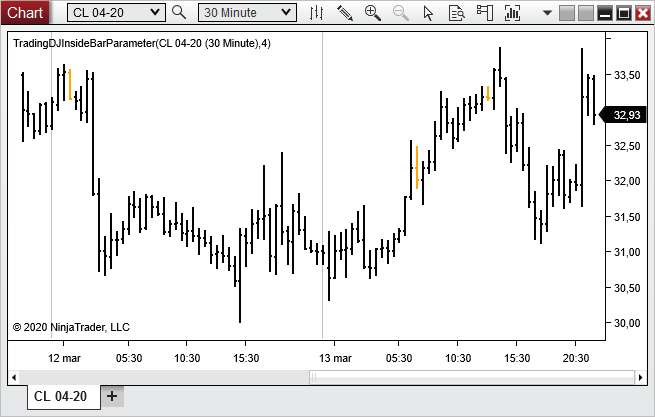
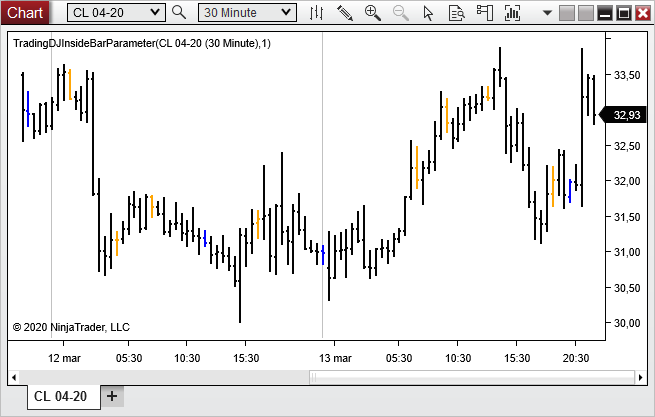
We could have gone a step further to allow to change the colors of the inside bar. All we had to do was to add two more parameters to our indicator that would generate the following code.
[NinjaScriptProperty]
[XmlIgnore]
[Display(Name="RisingBarsBrush", Description="Brush for the inside bar following consecutive rising bars", Order=1, GroupName="Parameters")]
public Brush RisingBarsBrush
{ get; set; }
[Browsable(false)]
public string RisingBarsBrushSerializable
{
get { return Serialize.BrushToString(RisingBarsBrush); }
set { RisingBarsBrush = Serialize.StringToBrush(value); }
}
[NinjaScriptProperty]
[XmlIgnore]
[Display(Name="FallingBarsBrush", Description="Brush for the inside bar following consecutive falling bars", Order=2, GroupName="Parameters")]
public Brush FallingBarsBrush
{ get; set; }
[Browsable(false)]
public string FallingBarsBrushSerializable
{
get { return Serialize.BrushToString(FallingBarsBrush); }
set { FallingBarsBrush = Serialize.StringToBrush(value); }
}
Then all we had to do was to assign it to BarBrush.
BarBrush = FallingBarsBrush;
//Insead of BarBrush = Brushes.Blue;
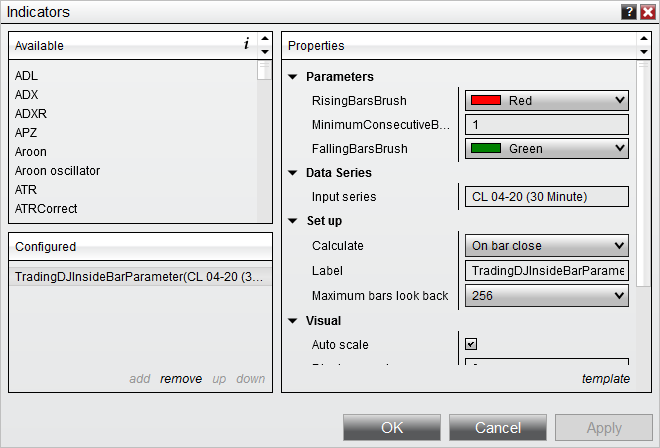
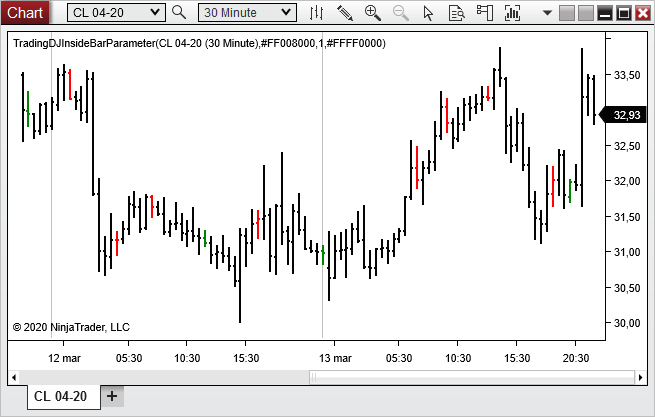
You can download the source code and the compiled version on GitHub.